What is Backbone Js?
Backbone is a lightweight library for structuring JavaScript code. It’s an MVC/MV * framework.
What is Backbone Js used for?
- Simplified Structure: Backbone.js provides a lightweight framework for building client-side web applications by organizing code into models, views, collections, and routers. This makes it easier to manage and maintain complex JavaScript code.
- Event-Driven Communication: It helps create interactive applications by offering event-driven communication between different components, reducing direct dependencies, and promoting better separation of concerns.
- Flexibility and Extensibility: Unlike more opinionated frameworks, Backbone.js is highly flexible. It allows developers to integrate it with existing codebases and extend them as needed, providing freedom in how the application is structured.
Backbone Js Components :
- Model: A model is the brain of your app—it’s where all the serious stuff happens. From grabbing data to updating it, models make sure everything’s running smoothly. What’s cool? When data changes, models can ping the view to update automatically, so users don’t have to refresh anything. Easy-peasy, right?
- View: Views are like your app’s front stage—it’s what your users actually see. They show data and respond to user actions (like clicks or typing). Tie them to models, and you get that sweet real-time feel where the screen updates instantly. Honestly, it’s magic for users.
- Collection:Handling a bunch of data? Collections are your best friend. They group models together, so you can sort, filter, and organize them without a headache. Plus, collections trigger their own events when things change, making everything run like clockwork.
- Router:Routers manage navigation, plain and simple. They listen to URL changes and make sure users land on the right page (or section) without the whole app reloading. For single-page apps, routers are a lifesaver—it’s how your app feels smooth and modern.
- Events: Think of events as the communication system of your app. They let different parts of your app talk to each other without stepping on toes. Need an example? When a button’s clicked, an event tells the rest of the app to react. It’s like a walkie-talkie for your code.
- Sync: Sync keeps your app and the server on the same page. Whether it’s fetching data, saving updates, or deleting stuff, sync makes sure the backend knows what’s going on. And yes, it’s all done with RESTful APIs (don’t worry, it sounds scarier than it is).
- History: History is the unsung hero of navigation. It keeps track of browser history and works with the router to handle URLs—whether they’re hash-based or fancy pushState ones. For users, it means seamless transitions and no weird reloads.
Model:
In Backbone.js, models are:
- Data Containers: Store data as key-value pairs, representing entities like users or products.
- Logic Handlers: Encapsulate business logic, including validation and transformations.
- Server-Syncing: Provide methods to fetch, save, and delete data on a server.
- Event-Driven: Trigger events on data changes, allowing views to update automatically.
Inheritance in Models:
In Backbone, you can create inheritance between models by extending existing models. This allows you to reuse and customize model logic, properties, and methods across different model types. Here’s how inheritance works in Backbone models:
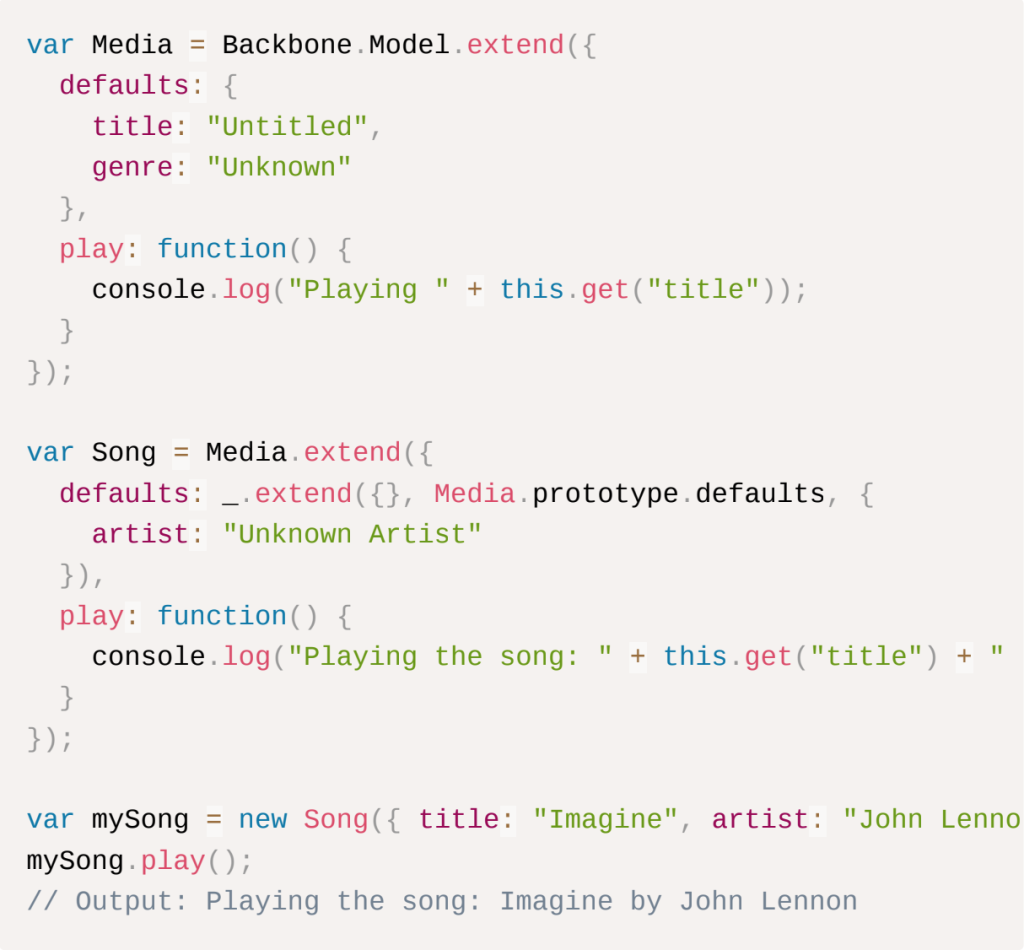
Views:
Understanding Views in Backbone.js
In Backbone.js, views are the bridge between your app’s data (models and collections) and what users see on the screen (the DOM). They handle rendering data and managing user interactions, making your app dynamic and user-friendly.
Think of views as the link that keeps your data and interface in sync. When the data changes, views automatically update what’s displayed—and when users interact, views can trigger updates to the data.
Key Features of Backbone Views
1. Rendering
Views control how data from models or collections is displayed in the DOM. Often, this involves using templates to create dynamic, reusable layouts that make development faster and cleaner.
2. Event Handling
Backbone views can listen for DOM events (like clicks, keypresses, or form submissions). When something happens, they trigger actions—like updating the model, changing the interface, or navigating to another part of the app.
3. Data Binding
Views are tightly connected to models and collections. They “listen” for events like change
or reset
, so whenever the data updates, the view knows to refresh automatically. No manual syncing—everything just works.
Why Backbone Views Are Crucial
Backbone views simplify the process of building interactive, user-focused applications by keeping your app’s interface and data in sync. Whether you’re creating a small widget or a full-featured app, views are your go-to tool for managing the connection between your data and the user experience.
Events:
In Backbone.js, events are pretty much the glue that holds your app together. They help different parts of your app communicate with each other when something changes, without you needing to do too much.
For instance, events can be triggered by things a user does, like clicking a button or typing something, or by changes in your data—like if a new model is added, or a collection gets updated. Backbone makes this process smoother, supporting both regular DOM events (handled through views) and custom events that you can create yourself.
Types of Events in Backbone
DOM Events in Views:
Views are your go-to for catching things like clicks or keypresses. Let’s say a user clicks a button, views catch that and react—maybe by updating the UI or triggering a data change. It’s pretty easy to set up.
Model and Collection Events:
Backbone’s models and collections can send out events too, whenever there’s a change in the data. So if a model gets updated, added, or removed, it sends a signal to the rest of your app. This keeps things in sync without you needing to manually refresh or check everything.
Custom Events:
Sometimes, the built-in events aren’t enough, and that’s where custom events come in. You can trigger custom events from any Backbone object—whether it’s a model, collection, or something else—and make other parts of your app respond. It gives you more flexibility when you need it.
Routers:
In Backbone.js, routers are like the traffic controllers of your app, handling all the navigation and URLs to make sure everything runs smoothly—kind of like how a single-page app should work.
Routers read parts of the URL and match them to specific actions. This way, you can navigate through your app—go back, forward, and all that—without having to reload the entire page. It’s what lets you have different “views” or “states” in your app without actually reloading every time.
Backbone.Router Overview
Defining Routes:
Routers let you set up URL patterns (or routes) and tie them to functions that handle what should happen when that route is hit. So, when someone visits a specific URL, Backbone knows what to do.
Listening to URL Changes:
Routers keep an eye on the URL, looking for changes. Whether it’s something like #profile/1 or a pushState change, they’ll detect it and make sure the app reacts correctly. No page reloads needed!
Executing Route Handlers:
Once the URL changes, Backbone matches it to one of the routes and runs the corresponding function. It’s pretty smooth and makes navigating your app feel fast and responsive.
Modularizing BackBone Application:
When you’re building a Backbone app, things can get pretty messy if you dont organize them right. Using RequireJS to modularize your app helps a ton. Instead of loading everything all at once, it lets you load just the parts you need when you need them. This makes the app faster and easier to deal with.
How to Modularize Your Backbone App with RequireJS
-
Set up RequireJS
First things first, you gotta get RequireJS up and running. It’s just setting it up so it knows where your modules are and how to load em when they’re needed. -
Define Modules for Backbone Parts
Then, you break up your app into different parts—like models, views, routers, collections—and make them separate modules. So, instead of loading your entire app at once, only the bits that are needed get pulled in. -
Start the App
After all that, you load the main module, and boom, your app starts running. This ties everything together and gets everything working.
How RequireJS Works in Backbone.js
- RequireJS: So, the
main.js
file loads theapp.js
thingy, which basically kicks everything off. - app.js: This is where the router gets set up and
Backbone.history
gets started up. - Router: The
AppRouter
defines routes, and those routes load up different views depending on the URL. - Views & Models: Each view and model is its own module, so only the stuff you actually need gets loaded when the app starts.